Custom service desks
Creating a custom service desk integration
Contents
Overview
Service desk requirements
Basic example
API Overview
API Details
Features by version
Supported response types
Agent app
File uploads
Overview
Web chat provides the ability to create a custom integration with a service desk or contact center that a user can use to communicate with a human agent. To create an integration, you will need to create an object or class that satisfies the web chat ServiceDesk interface for a service desk integration and then implement a factory function in the web chat configuration that will return a new instance of your integration when web chat asks for one.
The code for your integration will need to be included along with any code for your website or hosted separately. It cannot be hosted by Watson Assistant.
You can view our open source GitHub repository that contains a number of starter kits for various service desks. You can use these starter kits as a starting point for your integration or you can review them as examples for your own integration.
In order for web chat to integrate with a custom service desk, there are two basic steps that need to happen:
- Code must be written that communicates with the service desk (such as by starting a conversation or sending a message to a human agent) and satisfies the API contract defined by web chat.
- Web chat needs to be given access to that code using a factory function, so it can run it.
Service desk requirements
In order to create an integration between web chat and a service desk, the desk desk must support communication from a web browser and must be able to fulfill the web chat service desk API. This is usually accomplished using http endpoints provided by the service desk or a web sockets interface. In particular, the service desk must support the ability to start a chat, to receive messages from a user or to deliver messages from an agent to the user.
If the service desk requires calls to it to include secrets that must not be exposed to end users such as an API key, you will need to proxy the calls from web chat through middleware on your own server. This middleware would receive the calls from web chat and would forward them to the service desk along with the additional secret.
Unless the service desk is hosted on the same domain as your website, it will also need to support CORS in order to receive requests from a web browser. If the service desk does not support CORS, you can proxy the requests through your own server where CORS is not required.
Basic example
If you have implemented a service integration that satisfies the service desk API, getting web chat to use it only requires providing a factory function that can create a new instance of your integration. Below contains an example of an empty integration (that doesn't do any communicating with a service desk) to show how to register an integration with web chat. See the ServiceDeskFactoryParameters interface for details of the arguments that are passed to the function when it is called.
<script>
// Your custom service desk integration which can be located anywhere in your codebase.
class MyServiceDesk {
constructor(callback) {
this.callback = callback;
}
startChat() {
// This code will communicate with the SD to start the chat and is expected to eventually result in
// "callback.agentJoined" being called when an agent is available and "callback.sendMessageToUser"
// when the agent sends a message to the user.
// ...
}
endChat() {
// This code will communicate with the SD to tell it the chat was ended.
// ...
}
sendMessageToAgent() {
// This code will communicate with the SD to give the message from the user to an agent.
// ...
}
}
// Regular web chat embed script.
window.watsonAssistantChatOptions = {
integrationID: 'YOUR_INTEGRATION_ID',
region: 'YOUR_REGION',
serviceInstanceID: 'YOUR_SERVICE_INSTANCE_ID',
onLoad: function(instance) {
instance.render();
},
// **** The important part. This creates an instance of your integration.
serviceDeskFactory: (parameters) => new MyServiceDesk(parameters),
};
setTimeout(function(){const t=document.createElement('script');t.src='https://web-chat.global.assistant.watson.appdomain.cloud/versions/' + (window.watsonAssistantChatOptions.clientVersion || 'latest') + '/WatsonAssistantChatEntry.js';document.head.appendChild(t);});
</script>
API Overview
Web chat provides a public API that can be implemented by custom code that will enable web chat to communicate with a service desk in a manner where the communication is integrated into the web chat visual experience. The API provides functions such as startChat
to let the SD know when a chat should start and sendMessageToAgent
to send a message from the user to an agent. In addition, web chat provides a callback API that allows the SD to talk back to web chat. This includes functions like agendJoined
to let web chat know when an agent has joined the chat and sendMessageToUser
when the agent has sent a message to be displayed to the user.
Communicating from the web chat to your service desk
The serviceDeskFactory
configuration property expects a factory function that returns an object of functions or a class. This factory function and the parameters passed to it are documented in the TypeScript definitions. Web chat will call the class or functions returned from the factory as needed to communicate with your integration.
Communicating from your service desk to web chat
One of the items passed into the factory is a callback object. These callbacks are documented in the TypeScript definitions. These are the functions you will call inside your service desk code to communicate information back to the web chat.
Displaying the chat history to your human agent ("agent app")
Watson Assistant will pass configuration needed to display a chat history widget in your live agent application interface. This agent app will contain a copy of the conversation your customer had with Watson Assistant for your live agent to view. See the agent app section below for more info.
Interaction flow
Below is a list of the steps and actions that typically occur when a user is connected to a service desk and how web chat interacts with the service desk integration.
- When web chat is started, it will create a single instance of the service desk integration using the
serviceDeskFactory
configuration property. - A user sends a message to Watson Assistant and it returns a "Connect to Agent" response (response_type="connect_to_agent").
- If the service desk integration implements it, web chat will call
areAnyAgentsOnline
to determine if any agents are online. This determines whether web chat displays a "request agent" button or if it shows the "no agents available" message instead. - User clicks the "request agent" button.
- Web chat will call the
startChat
function on the integration. The integration should ask the service desk to start a new chat. - A banner is displayed to the user to indicate web chat is connecting them to an agent.
- If the service desk provides the capability, the integration may call
callback.updateAgentAvailability
to update the banner letting the user know how long the wait might be. - When an agent becomes available, the integration should call
callback.agentJoined
and web chat will inform the user that an agent has joined. - When an agent sends a message, the integration should call
callback.sendMessageToUser
. - When the user sends a message, web chat will call
sendMessageToAgent
. - The user ends the chat.
- Web chat will call
endChat
on the integration. This will tell the service desk that the chat is over.
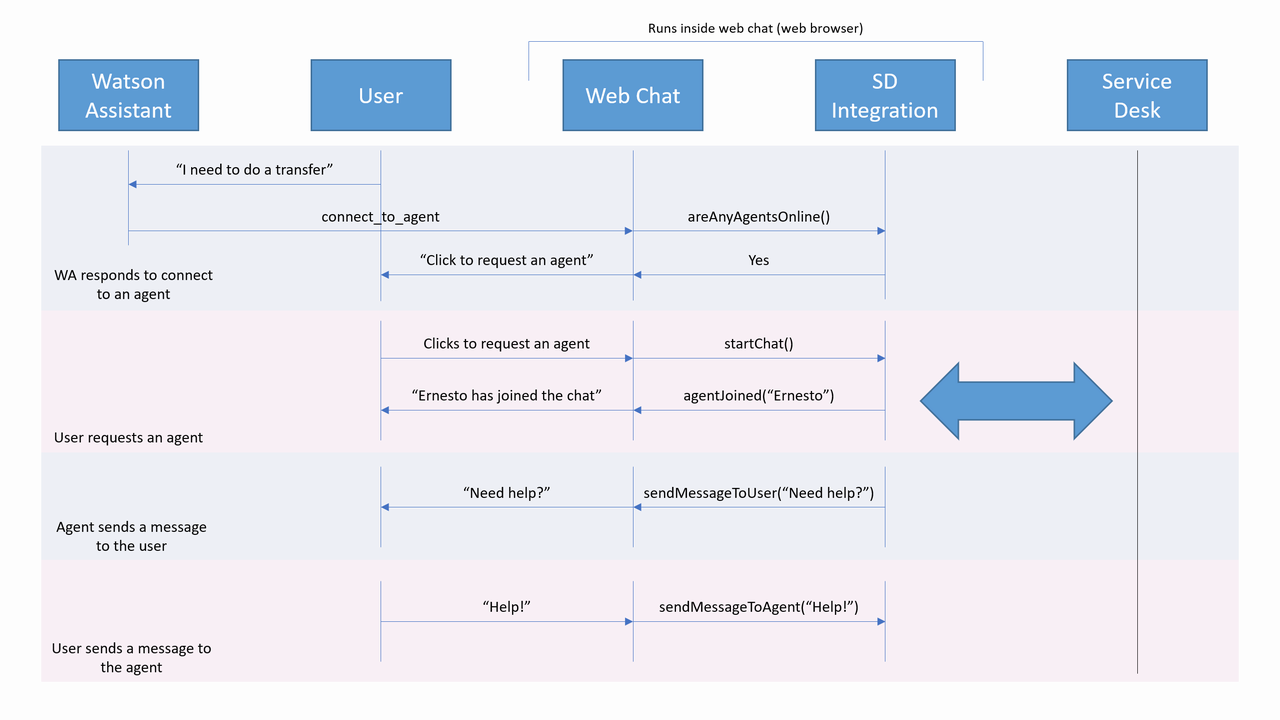
API Details
Below is a quick list of the methods used by a custom service desk integration. You can find detailed documentation for these functions by looking at the TypeScript interfaces that we have defined for them.
The following methods from the ServiceDesk interface should be implemented by your integration.
- getName
- startChat
- endChat
- sendMessageToAgent
- updateState (optional)
- userReadMessages (optional)
- areAnyAgentsOnline (optional)
The following methods from the ServiceDeskCallback interface are available that can be called by your integration.
- agentEndedChat
- agentJoined
- agentLeftChat
- agentReadMessages
- agentTyping
- beginTransferToAnotherAgent
- sendMessageToUser
- setErrorStatus
- setFileUploadStatus
- updateAgentAvailability
- updateCapabilities
Features by version
If you are building a custom service desk to be used by others who may not be using the same version of web chat, you need to pay attention to which features of web chat will be available to your integration. You can check the version of web chat being used by calling instance.getWidgetVersion. Beginning with web chat 7.1.0, the instance object will be available on the service desk factory params object. You can use the semver-compare library to compare versions. Your integration should either throw an error or respond gracefully if a feature it wants to use is not available in the version of web chat that is loaded.
Below is a list of feature changes that have been made to web chat by version.
5.1.1
- Web chat added support for the
userTyping
integration function.
6.7.0
- A
AgentAvailability.message
property was added allowing a service desk to report a custom message to the user who is waiting for an agent to join. - A
ConnectingErrorInfo.messageToUser
property was added allowing a service desk to report a custom error message to the user when there is a problem connecting to a service desk. - The
sendMessageToUser
function may now be passed just a string in addition to aMessageResponse
object.
7.1.0
- A
ServiceDeskFactoryParameters.instance
property was added giving the service desk access to the running instance of web chat. - Added support for custom responses.
Supported response types
The callback.sendMessageToUser
function lets your integration display a message to the user. The function allows you to provide a simple string which will display a basic text response to the user. In addition, this function allows you to provide a Watson Assistant v2 API MessageResponse object. When receiving messages from an agent, web chat only supports a subset of the response types that are supported when receiving messages from Watson Assistant. Below is a list of the Watson Assistant response types that are supported from a service desk integration. Note that web chat does support markdown in text responses.
- text
- image
- video
- custom (use the customResponse event for these)
Additional response types
In addition to the Watson Assistant response types that are supported, there are a number of web chat specific response types that are also supported that your integration may use. These are listed below.
inline_error
This response type will display an error message to the user.
const message = {
output: {
generic: [
{
response_type: 'inline_error',
text: 'An error has occurred',
}
],
},
};
callback.sendMessageToUser(message, agent.id);
button
This response type allows you to display a clickable link to the user. At the moment, the link
button type is the only supported type. This response type may be useful if the agent has sent a file to the user to be downloaded.
const message = {
output: {
generic: [
{
response_type: 'button',
style: 'link',
type: 'url',
url: 'https://placekitten.com/400/300',
label: 'Kitten1.png',
}
],
},
};
callback.sendMessageToUser(message, agent.id);
Agent app
The agent app is a special version of web chat that runs inside the UI of your service desk. It will contain a copy of the conversation your customer had with Watson Assistant for your live agent to view.
When the startChat
function of your service desk integration is called, it will contain a startChatOptions.agentAppInfo.sessionHistoryKey
property. This property is a string that contains everything web chat needs to securely open a read-only mode of the web chat showing a transcript of the conversation between the end user and Watson Assistant. The vast majority of service desks have a way to pass metadata or custom attributes to the agent's view and either embed an iFrame or embed HTML and JavaScript. It is up to you to determine how to pass the sessionHistoryKey to either the IBM provided iFrame or JavaScript.
Viewing agent application via iFrame
If your service desk allows you to create insert an iFrame into the agent's UI, you would do so by providing a URL like the following:
https://web-chat.global.assistant.watson.appdomain.cloud/loadAgentAppFrame.html?session_history_key=INSERT_KEY_HERE
Viewing agent application via HTML/JavaScript
Alternatively, you can load in a JavaScript file that you can feed the sessionHistoryKey to.
<div id="agent-app-host" style="width: 380px; height: 380px;"></div>
<script src="https://web-chat.global.assistant.watson.appdomain.cloud/loadAgentApp.js" />
</script>
File uploads Beta
Web chat supports the ability to allow users to select local files to upload to an agent. To use this functionality, your integration will need to perform the following steps. Refer to the TypeScript interfacesfor complete details on the functions being referred to here. This feature is currently in beta. The text for the feature is only available in English.
Enable file uploads
The first step is for your service desk to tell web chat that it supports file uploads using the callback.updateCapabilities
function. It can also tell web chat if it supports multiple files and what filter to apply to the operating system file select dialog. This function can be called at any time and it may be called to change the current capabilities. For example, if your service desk does not allow files to be uploaded until an agent has requested files from the user, you may wait to call this function until the user has received such a message from the agent.
this.callback.updateCapabilities({
allowFileUploads: true,
allowedFileUploadTypes: 'image/*,.txt',
allowMultipleFileUploads: true,
});
Validating files before uploading
When a user selects files to be uploaded, web chat will call the filesSelectedForUpload
function on your integration. You can use this function to validate that the file is appropriate for uploading. You can check the file's type or file size and report an error if it is not valid. The user must remove any files that are in error before sending a message with the files. You can report the error by calling the callback.setFileUploadStatus
function.
filesSelectedForUpload(uploads: FileUpload[]): void {
uploads.forEach(upload => {
if (upload.file.size > this.maxFileSizeKB * 1024) {
const maxSize = `${this.maxFileSizeKB}KB`;
const errorMessage = this.instance.getIntl().formatMessage({ id: 'fileSharing_fileTooLarge' }, { maxSize });
this.callback.setFileUploadStatus(upload.id, true, errorMessage);
}
});
}
Handling uploaded files
After you have enabled file uploads from the user. The user may then select files and upload them to an agent. This will occur by the user sending the files as a "message". The files will be passed to your integration when the sendMessageToAgent
function is called. The files will be passed as the additionalData.filesToUpload
argument. Note, that the user is not required to type a message so the message.input.text
value might be empty.
Once your integration has received the files to upload, it should perform whatever operations are necessary to actually send the files to the service desk.
async sendMessageToAgent(message: MessageRequest, messageID: string, additionalData: AdditionalDataToAgent) {
if (message.input.text) {
// Send the user's text input to the service desk.
// ...
}
if (additionalData.filesToUpload.length) {
// Execute whatever operation is necessary to send the files to the service desk.
// ...
}
}
Updating file upload status
Once a file has finished uploading either because it was completed successfully or was stopped due to an error, your integration should report the status to the user using the callback.setFileUploadStatus
function.
// Called when the service desk has reported an error.
this.callback.setFileUploadStatus(file.id, true, errorMessage)